# Manually call API
# Configure callback URL
You need to set a callback link (Callback URL) on the configuration page of social login, and the user will be redirected to the callback address after logging in:
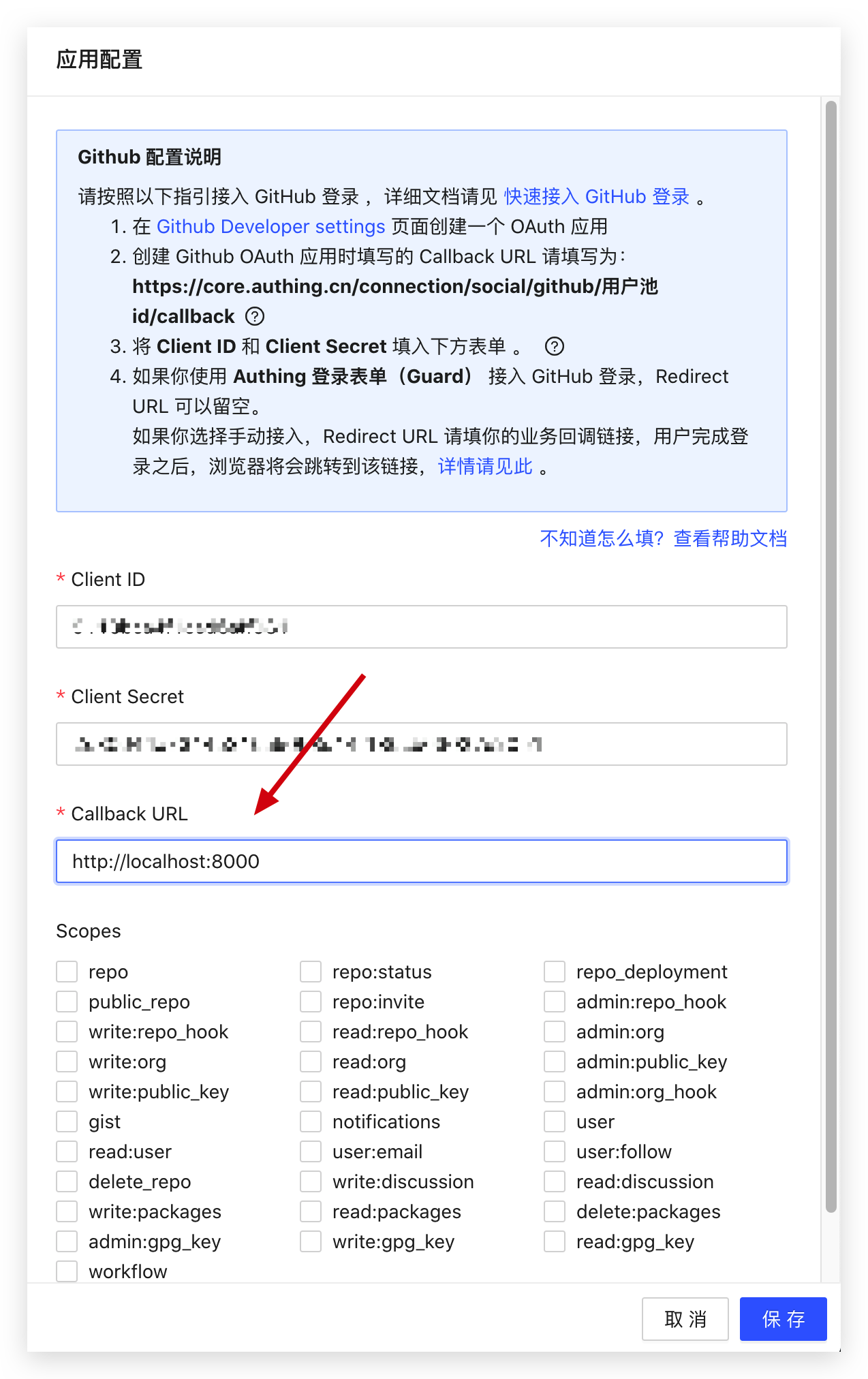
# Guide users to the social login authorization page
This method is only applicable to social login on the Web side. If you need to initiate a social login in the mobile App, please read the documentation of the relevant social login service provider.
This operation should be done in the browser. You can place a clickable button or Logo link on the Web page to the link above so that users can click to log in.
The following is the authorization page link corresponding to each web social login, where YOUR_APP_ID
is your application ID (note not the user pool ID). You can view the application ID on the application list and application details page.
Social login method | Social login authorization link |
---|---|
GitHub (opens new window) | https://core.authing.cn/connection/social/github?app_id=<YOUR_APP_ID> |
WeChat PC Scan Code (opens new window) | https://core.authing.cn/connection/social/ wechat:pc?app_id=<YOUR_APP_ID> |
WeChat webpage authorization (opens new window) | https://core.authing.cn/connection/social/wechat:webpage-authorization ?app_id=<YOUR_APP_ID> |
Enterprise WeChat internal application scan code login (opens new window) | https://core.authing.cn/connection/social/wechatwork :corp:qrconnect?app_id=<YOUR_APP_ID> |
Enterprise WeChat third-party application scan code login (opens new window) | https://core.authing.cn/connection/social/ wechatwork:service-provider:qrconnect?app_id=<YOUR_APP_ID> |
DingTalk (opens new window) | https://core.authing.cn/connection/social/dingtalk?app_id=<YOUR_APP_ID> |
Sina Weibo (opens new window) | https://core.authing.cn/connection/social/weibo?app_id=<YOUR_APP_ID> |
QQ (opens new window) | https://core.authing.cn/connection/social/qq?app_id=<YOUR_APP_ID> |
Google (opens new window) | https://core.authing.cn/connection/social/google?app_id=<YOUR_APP_ID> |
Alipay (opens new window) | https://core.authing.cn/connection/social/alipay?app_id=<YOUR_APP_ID> |
Sign in with Apple (opens new window) | https://core.authing.cn/connection/social/apple:web?app_id=< YOUR_APP_ID> |
# Processing Authing callback request
After the user agrees to the authorization in the previous step, it will jump to the Authing server first, and then Authing will carry the user information to jump to the business callback link configured by the developer in the Authing console, with the following Get request parameters:
Parameters | Description |
---|---|
code | Error or success code, 200 is success, non-200 is failure |
message | Success or error message |
data | userInfo, if code is not 200, this parameter is not returned |
Some browsers and Web Servers may have a 404 when the URL is too long, such as ASP.NET. At this time, you need to modify the configuration. For details, see [This StackOverflow Answer](https://stackoverflow.com/questions/ 28681366/in-asp-net-mvc-would-a-querystring-too-long-result-in-404-file-not-found-error/28681600).
The following is the code that uses JavaScript to get user data from URL parameters:
// Get URL parameters
function getQueryString(name) {
var reg = new RegExp("(^|&)" + name + "=([^&]*)(&|$)", "i");
var r = window.location.search.substr(1).match(reg);
if (r != null) {
return unescape(r[2]);
}
return null;
}
// Convert Code to Int type to facilitate judgment
const code = parseInt(getQueryString("code"));
if (code !== 200) {
// error
const errorMsg = getQueryString("message");
// Show errorMsg to users or perform other business...
} else {
const userInfo = getQueryString("data");
// Store token in localStorage
// It is recommended to attach Token to subsequent requests and verify the validity of Token by the backend
localStorage.setItem("token", userInfo.token);
}
# Next
After obtaining the user information, you can get the login credential token. You can carry this token in subsequent API requests, and then distinguish different users based on this token in the backend interface. For details, see [Verification token](../ ../advanced/verify-jwt-token.md#yan-zheng-authing-qian-fa-de-token).